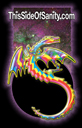 music |
 | | OSdata.com |
declarations
summary
This subchapter looks at declaring variables.
Professors are invited to give feedback on both the proposed contents and the propsed order of this text book. Send commentary to Milo, PO Box 1361, Tustin, California, 92781, USA.
declarations
This subchapter looks at declaring variables.
variable identifiers
Variables are named with identifiers. In most languages variables may be given any valid identifer name.
Variables may have any valid identifier name in C.
Variables may have any valid identifier name in Pascal.
Variables may have any valid identifier name in PL/I.
explicit and implied declaration
Some languages require that variables be explicitly declared, while other languages allow an implicit declaration.
Variables should be explicitly declared by type in C. Variables without a type specification default to extern int.
Variables must be explicitly declared in Pascal.
Undeclared variables that start with the letters I through N, inclusive, (short for INteger) are assumed to be integers, while all other undeclared variables are assumed to be floating point.
Undeclared variables that start with the letters I through N, inclusive, (short for INteger) are assumed to be integers, while all other undeclared variables are assumed to be floating point.
declaration location
Some languages require that variables be declared in a specific portion of the program, while others allow variable declarations to be scattered through a program (but usually still must be before first actual use).
C variables may appear in any of several recommended locations. Variables declared in unusal locations may exhibit strange or unpredicatable behavior. Recmmended locations include the beginnings of source files or translation units, beginnings of functions, and beginnings of blocks.
Pascal variables must be declared in the declaration part, which comes immediately before the statement part.
program SimpleProgram (output);
var Age: Integer;
begin
Age := 21;
write ('Susan is ');
write (Age);
writeln (' years old.')
end.
Note that outputting a variable involves giving the name of the variable without any apostrophes (quotation marks).
The output of this program would be:
Susan is 21 years old.
end.
21 The body of a program unit generally contains two parts: a declarative part, which defines the logical entities to be used in the program unit, and a sequence of statements, which defines the execution of the program unit. :Ada-Europes Ada Reference Manual: Introduction: Language Summary See legal information
22 The declarative part associates names with declared entities. For example, a name may denote a type, a constant, a variable, or an exception. A declarative part also introduces the names and parameters of other nested subprograms, packages, task units, protected units, and generic units to be used in the program unit. :Ada-Europes Ada Reference Manual: Introduction: Language Summary See legal information
declaration format
The format for a declaration varies by programming language.
In C the order of the parts of a declaration are: a list of storage class specifiers (such as register), type specifiers (such as int), and type qualifiers (such as const), a list of names of variables, an optional equal sign and initialization value, and the semicolon separator.
extern int age = 21;
In Pascal the order of the parts of a declaration are: the reserved word var, the name of the variable, a colon character ( : ), the type of the variable, and the semicolon terminator.
var Age: Integer;
The following material is from the unclassified Computer Programming Manual for the JOVIAL (J73) Language, RADC-TR-81-143, Final Technical Report of June 1981.
1.1.2 Storage
When a JOVIAL program is executed, each value it operates on is
stored as an item. The item has a name, which is declared and
then used in the program when the value of the item is fetched or
modified.
An item is declared by a JOVIAL statement called a declaration
statement. The declaration provides the compiler with the
information it needs to allocate and access the storage for the
item. Here is a statement that declares an integer item:
ITEM COUNT U 10;
This declaration says that the value of COUNT is an integer that
is stored without a sign in ten or more bits. The notation is
compact: "U" means it is an unsigned integer, "10" means it
requires at least 10 bits. We say "at least" then bits because
the JOVIAL compiler may allocate more than ten bits. (That
allocation wastes a little data space, but can result in faster,
more compact code.)
Chapter 1 INTRODUCTION, page 3
JOVIAL does not require that you give the number of bits in the
declaration of an integer item. If you omit it, JOVIAL supplies
a default value that depends on which implementation of JOVIAL
you are using. An example is:
ITEM TIME S;
This statement declares TIME to be the name of an integer
variable item that is signed and has the default number of bits.
On one implementation of JOVIAL, this would be equivalent to the
declaration:
ITEM TIME S 15;
The item TIME occupies 16 bits (including the sign). On another
implementation, it would be equivalent to:
ITEM TIME S 31;
This and other defaults are defined in the user"s manual for the
implementation of JOVIAL you are using.
In this brief introduction, we cannot consider each kind of item
in detail (as we just did for integer items). Instead, a list of
examples follow, one declaration for each kind of value.
ITEM SIGNAL S 2; A signed integer item, which occupies
at least three bits and accomodates
values from -3 to +3.
ITEM SPEED F 30; A floating item, whose value is stored
as a variable coefficient (mantissa)
and variable scale factor (exponent).
The "30" specifies thirty bits for the
mantissa and thus determines the
accuacy of the value. The number of
bits in the exponent is specified by
the implementation, not the program.
It is always sufficient to accommodate
a wide range of numbers.
ITEM ANGLE A 2,13; A fixed item, whose value is stored
with fixed scaling, namely two bits to
the left of the binary point and
thirteen fractional bits. Thus it
accomodate a value in the range -4 <
value < +4 to a precision of
1/(2**14).
Chapter 1 INTRODUCTION, page 4
ITEM CONTROLS B 10; A bit-string item, whose value is a
sequence of ten bits. Thus it can
accommodate, for example, the settings
of ten on/off console switches.
ITEM MESSAGE C 80; A character-string item, whose value
is a sequence of eighty characters.
This it can accommodate, for example,
the message "WARNING: Cooling system
failure" (with plenty of character
positions left over).
ITEM INDICATOR STAUS (V(RED),V(YELLOW),V(GREEN));
A status item, whose value can be
thought of as "V(RED)", "V(YELLOW)",
or "V(GREEN)" but which is, in fact,
compactly stored as an integer. Thus
a programmer culd assign "V(RED)" to a
variable to indicate cooling system
failure instead of using a (presumably
non-mnemonic) integer.
ITEM HEAD P DATA; A pointer item, whose value is the
address of a data object of type DATA.
Items are just the scalar (single-value) data of JOVIAL. JOVIAL
also has tables and blocks, which provide for arrays and other
data structures.
An example of a table declaration is:
TABLE GRID (1:10, 1:10);
BEGIN
ITEM XOORD U;
ITEM YCOORD U;
END
The table GRID has two dimensions. Each dimension contains ten
entries. Each entry consists of two items, XCOORD and YCOORD.
An example of a block declaration is:
BLOCK GROUP;
BEGIN
ITEM FLAG B;
TABLE DATA(100);
ITEM POINT U;
END
The block GROUP contains the item FLAG and the table DATA.
Chapter 1 INTRODUCTION, page 5
Items, tables, and blocks can also be declared using type-names.
A type-name is defined in a type declaration. An example of a
type declaration is:
TYPE COUNTER U 10;
The type-name COUNTER can be used to declare ten-bit integers.
For example:
ITEM CLOCK COUNTER;
Chapter 1 INTRODUCTION, page 6
multiple declarations
In almost all languages each variable may be declared on a separate line.
In many languages it is possible to declare several variables of the same type in a single declaration.
In C it is possible to declare multiple variables in the same declaration as long as they all share the exact same specifiers and qualifiers. In this case the variable identifier names will be separated by a comma.
int RunningSubTotal, FinalTotal
In Pascal it is possible to declare multiple variables in the same declaration as long as they all share the exact same type. In this case the variable identifier names will be separated by a comma.
var RunningSubTotal, FinalTotal: integer;
free music player coding example
Coding example: I am making heavily documented and explained open source code for a method to play music for free almost any song, no subscription fees, no download costs, no advertisements, all completely legal. This is done by building a front-end to YouTube (which checks the copyright permissions for you).
View music player in action: www.musicinpublic.com/.
Create your own copy from the original source code/ (presented for learning programming).
Because I no longer have the computer and software to make PDFs, the book is available as an HTML file, which you can convert into a PDF.
Names and logos of various OSs are trademarks of their respective owners.