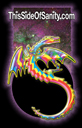 music |
 | | OSdata.com |
Hello World
summary
The educational goal of this subchapter is to give the student a general idea of what a simple computer program looks like in their chosen language. The professor may present only the example from the programming language being taught or may show many examples to give a feel for some of the similarities and differences between common programming languages.
The classic example for any programming language is Hello World (a simple program that writes the text Hello World). These examples show how to write this simple program in different programming languages.
Hello World
The cliche first program is Hello World a simple program that types the message Hello World. Even experienced programmers will often write a simple Hello World program when learning a new programming language or switching to a new development environment.
Kernighan and Ritchie popularized the Hello World! program in their book C Programming Language.
Every programming language has its own peculair rules for the form of a program. The Hello World program is a fine illustration of the basic rules of a programming language.
All of the code below produces the same basic results: printing or typing the phrase Hello World. You may want to take a brief look at how different languages can have very different methods for achieving the same results.
with Ada.Text_IO;
use Ada.Text_IO;
procedure HelloWorld is
begin
Ada.Text_IO.Put_Line("Hello World");
end HelloWorld;
Ada was first released in 1983 (ADA 83), with major releases in 1995 (ADA 95) and 2005 (ADA 2005). Ada was created by the U.S. Department of Defense (DoD), originally intended for embedded systems and later intended for all military computing purposes.
Ada is named for Augusta Ada King, the Countess of Lovelace, the first computer programmer in modern times.
BEGIN
OUTSTRING(2, "Hello World");
END.
ALGOL (ALGOrithmic Language) was first formalized in 1958 (ALGOL 58), with major releases in 1960 (ALGOL 60) and 1968 (ALGOL 68). ALGOL was originally intended for scientific computations.
ALGOL is considered to be the first second generation computer language.
ALGOL was a highly influential programming language. Most modern programming languages are descendants of ALGOL.
ALGOL introduced such concepts as: block structure of code, scope of variables, BNF notation for defining syntax, dynamic arrays, reserved words, and user defined data types.
10 PRINT "Hello World"
BASIC (Beginners All-purpose Symbolic Instruction Code) was designed as a teaching language in 1963 by John George Kemeny and Thomas Eugene Kurtz of Dartmouth College.
#include <stdio.h>
main()
{
printf("Hello World\n");
}
C was developed in 1972 by Dennis Ritchie of Bell Telephone Laboratories for use in systems programming for UNIX.
#include <iostream.h>
int main(int argc, char *argv[])
{
cout << "Hello World" << endl;
return 0;
}
C++ was developed in 1983 by Bjarne Stroustrup at Bell Telephone Laboratories to extend C for object oriented programming.
IDENTIFICATION DIVISION.
PROGRAN-ID. HelloWorld.
AUTHOR. Milo.
ENVIRONMENT DIVISION.
CONFIGURATION SECTION.
INPUT-OUTPUT SECTION.
DATA DIVISION.
FILE SECTION.
WORKING-STORAGE SECTION.
LINKAGE SECTION.
PROCEDURE DIVISION.
DISPLAY "Hello World".
STOP RUN.
COBOL (COmmon Business Oriented Language) was created in 1959 by the Short Range Committee of the U.S. Department of Defense (DoD). Official ANSI standards included COBOL-68 (1968), COBOL-74 (1974), COBOL-85 (1985), and COBOL-2002 (2002). COBOL 97 (1997) introduced an object oriented version of COBOL.
define method hello-world()
format-out("Hello World\n");
end method hello-world;
hello-world();
Dylan was created in the early 1990s by Apple Computer and others. Dylan was originally intended for use with the Apple Newton, but wasnt finished in time.
: hello_world ." Hello World" ;
Forth was created by Charles H. Moore in 1968. Forth was a reference to Moores claim that he had created a fourth generation programming language.
PROGRAM HELLO
WRITE(UNIT=*, FMT=*) 'Hello World'
END
FORTRAN (FORmula TRANslation) was created in 1954 at International Business Machines (now IBM). FORTRAN is the oldest programming language still in common use.
<html>
<head>
<title>Hello World</title>
</head>
<body>
<p>Hello World</p>
</body>
</html>
HTML (HyperText Markup Language) was developed in 1989.
import java.applet.*;
import java.awt.*;
Public class HelloWorld extends Applet
{
public void paint(Graphics g)
{
g.drawstring("Hello World".,10,10);
}
}
Java (a reference to coffee) was created by Sun Microsystems and released in 1995.
(print "Hello World".)
LISP (LISt Processing) was created n 1958 by John McCarthy of MIT. LISP is the second oldest programming language still in common use.
print [Hello World]
Logo was created in 1967 by Daniel G. Bobrow, Wally Feurzeig, and Seymour Papert.
MODULE HelloWorld;
FROM InOut IMPORT WriteString, WriteLn;
BEGIN
WriteString('Hello World');
WriteLn;
END HelloWorld.
Modula (MODUlar LAnguage) was created by Niklaus Wirth, who started work in 1977. Modula-2 was released in 1980.
MODULE HelloWorld;
IMPORT Out;
BEGIN
Out.Open;
Out.String('Hello World');
END HelloWorld.
Oberon (named for a moon of Uranus) was created in 1986 by Niklaus Wirth.
PROGRAM HelloWorld (OUTPUT);
BEGIN
WRITELN('Hello World');
END.
Pascal (named for French religious fanatic and mathematician Blaise Pascal) was created in 1970 by Niklaus Wirth.
print "Hello World\n";
Perl (Practical Extracting and Report Language) was created by Larry Wall in 1987.
<?php
echo "Hello World\n";
?>
PHP (PHP Hypertext Processor) was created by Rasmus Lerdorf in 1995.
HELLO: PROCEDURE OPTIONS (MAIN);
PUT SKIP LIST('HELLO WORLD');
END HELLO;
PL/I (Programming Language One) was created in 1964 at IBMs Hursley Laboratories in the United Kingdom.
/Courier findfont
14 scalefont
setfont
0 0 moveto
(Hello World) show
showpage
PostScript was created in 1984 by John Warnock and Chuck Geschke at Adobe.
?- write('Hello World'), nl.
Prolog (PROgramming LOGic) was created in 1972 by Alan Colmerauer with Philippe Roussel.
For Python 2.6 and earlier:
print: "Hello World"
For Python 3.0 and later:
print("Hello World")
Python (named for Monty Python Flying Circus) was created in 1991 by Guido van Rossum.
"Hello World\n".display
Ruby was created in 1995 by Yukihiro Matsumoto.
'Hello World' out.
SmallTalk was created in 1969 at Xerox PARC by a team led by Alan Kay, Adele Goldberg, Ted Kaehler, and Scott Wallace.
OUTPUT = "Hello World"
END
SNOBOL (StroNg Oriented symBOli Language) was created in 1962.
SELECT "Hello World"
SQL (Standard Query Language) was designed by Donald D. Chamberlin and Raymond F. Boyce of IBM in 1974.
other
26. There will always be things we wish to say in our programs that in all known languages can only be said poorly. Alan Perlis, Epigrams on Programming, ACMs SIGPLAN Notices Volume 17, No. 9, September 1982, pages 7-13
free music player coding example
Coding example: I am making heavily documented and explained open source code for a method to play music for free almost any song, no subscription fees, no download costs, no advertisements, all completely legal. This is done by building a front-end to YouTube (which checks the copyright permissions for you).
View music player in action: www.musicinpublic.com/.
Create your own copy from the original source code/ (presented for learning programming).
Because I no longer have the computer and software to make PDFs, the book is available as an HTML file, which you can convert into a PDF.
Names and logos of various OSs are trademarks of their respective owners.