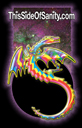 music |
 | | OSdata.com |
simple music player code example
summary
This is an example program for teaching computer programming. The example is a free music player, completely legal, that does not have any subscription fees, download costs, or advertisements.
To support the still unfinished college text book on computer programming (table of contents), I am posting heavily commented and described open source code for a free music player. A working example (still very new and under construction) is at www.thissideofsanity.com/musicbox/musicbox.php. This source code is free for any legal non-commercial and/or non-profit and/or educational and/or private purpose.
The project starts with a very simple music player (simplified from my first experiments in this area).
If you dont have access to a remote web server (at school or under your own account), you can install a working Apache web server, MySQL data base, and PHP scripting language and run this entire programming project from your local computer in your favorite web browser. See local server.
license
This is example code from This Side of Sanity, released under Apache License 2.0.
Copyright 2012, 2013, 2014 Milo
Licensed under the Apache License, Version 2.0 (the License); you may not use this file except in compliance with the License. You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an AS IS BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions and limitations under the License.
summary
This is an example program for teaching computer programming. The example is a free music player, completely legal, that does not have any subscription fees, download costs, or advertisements.
To support the still unfinished college text book on computer programming (table of contents), I am posting heavily commented and described open source code for a free music player. A working example (still very new and under construction) is at www.thissideofsanity.com/musicbox/musicbox.php. This source code is free for any legal non-commercial and/or non-profit and/or educational and/or private purpose.
The project starts with a very simple music player (simplified from my first experiments in this area).
If you dont have access to a remote web server (at school or under your own account), you can install a working Apache web server, MySQL data base, and PHP scripting language and run this entire programming project from your local computer in your favorite web browser. See local server.
components used
Download the following components to your hard drive, rename the file simple.txt to simple.php, and then upload all of the items to your server (or schools server - with permission from your school) or set up a free Apache server on your local machine.
start of file
The file starts with a fairly standard HTML doctype and html tag. You may use any doctype you choose. I use the transitional to allow a combination of new and old HTML. You may change the language to any language you desire.
These items are outputted to the web browser.
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN">
<html lang="en" dir="ltr">
start of php
This is one of the ways to indicate that the file has switched from standard HTML to PHP code.
<!?php
globals
There are no declared global variables in this example.
/******************************/
/* GLOBALS */
/******************************/
functions
There are no declared functions in this example.
/******************************/
/* FUNCTIONS */
/******************************/
main section
This is where the actual PHP program starts.
/******************************/
/* MAIN PAGE */
/******************************/
GET test
This block of code checks the URL to see if there is information passed on from the previous song played. This uses the GET method.
The empty
built-in PHP function tests to see if there is anything in the $_GET
built-in variable.
If the URL contains no information for us, we set the variable $lastsong
to zero to indicate this (zero is never a valid song number). This is usually the case of the first use of the simple player (or a return via link or bookmark).
If the URL contains the previous song played, we store this information in our variable $lastsong
.
This is used later to prevent the simple player from playing the same song twice in a row.
if(empty($_GET))
$lastsong = 0;
else
$lastsong = $_GET['lastsong'];
randomize
We get a random number between one and four, inclusive.
We have four songs loaded up as examples:
- 2011 Grammy song of the year: Rolling In The Deep by Adele
- 2011 Grammy rock song: Walk by the Foo Fighters
- 2011 Grammy rap song: All Of The Lights by Kanye West, Rihanna, Kid Cudi, and Fergi
- 2011 Grammy country song: Barton Hollow by the Civil Wars
You should change these to songs of your own choosing.
$songnumber = rand(1,4);
check for repeats
We use a while
loop to force the songs to never repeat.
We keep comparing a random number to the last song until they are different.
In the special case of the first use of the player, the last song is set to zero, which never matches any valid song number.
while ( $songnumber == $lastsong )
{
$songnumber = rand(1,4);
}
set song information
Now that we know which song is to be played, we set the song information.
This particular method will only work for a fairly small number of songs before becoming cumbersome (probably around a hundred or so songs). And at some point it will take so much time that you run into the limit on how long a script can run. Our more advanced example will use an SQL data base to store song information.
We use the switch
statement to fetch the correct song information, based on the $songnumber
.
The default
case is provided just in case we made a mistake (left out a number or some other typing error). This way a song will still play.
Character strings are in single quotes ('
) because we dont interpret anything inside of them.
switch($songnumber)
{
case 1 :
$youtubecode = 'eLEX82mLnCM';
$songlength = '236';
$songtitle = 'Rolling In The Deep';
$songartist = 'Adele';
$description = 'This was the 2011 grammy song of the year.';
break;
case 2 :
$youtubecode = '4PkcfQtibmU';
$songlength = '359';
$songtitle = 'Walk';
$songartist = 'the Foo Fighters';
$description = 'This was the 2011 Grammy rock song.';
break;
case 3 :
$youtubecode = 'jjDdGKBhTuo';
$songlength = '302';
$songtitle = 'All of the Lights';
$songartist = 'Kanye West, Rihanna, Kid Cudi, and Fergi';
$description = 'This was the 2011 Grammy rap song.';
break;
case 4 :
$youtubecode = 'ODOOo-R6kg8';
$songlength = '233';
$songtitle = 'Barton Hollow';
$songartist = 'the Civil Wars';
$description = 'This was the 2011 Grammy country song.';
break;
default :
$youtubecode = 'eLEX82mLnCM';
$songlength = '236';
$songtitle = 'Rolling In The Deep';
$songartist = 'Adele';
$description = 'This was the 2011 grammy song of the year.';
break;
}
adjust time
We stored the length of each video in seconds.
We add five more seconds as a fudge factor for download and buffering times.
$songlength = $songlength +5;
refresh command
We use a really old HTML command to force the web page to refresh after the indicated number of seconds (which happens to match the time we want the current music video to play).
We also adjust the URL so that the GET
method will include the previous song played (to avoid repeats).
Note that you need to change the URL=
to match the location where your copy of the music player is running (either on an actual web site or from local host on your own computer).
Strings use double quotes ("
) because we use escape characters and want those interpretted.
echo "<head><title>Personal Music Box"</title>\n";
echo "<META HTTP-EQUIV=\"Refresh\"
CONTENT=\"".$songlength."; URL=http://www.osdata.com/music/code/simple.php?lastsong=".$songnumber."\">\n";
Switch to HTML for head
We switch back to HTML output to deliver the head and the start of the body.
?>
<meta HTTP-EQUIV="Content-Type" CONTENT="text/html; charset=ISO-8859-1">
-rest of head-
<body text="#000000" bgcolor="#ccccff">
-more HTML code-
identify music video
We provide the name of the song and artist to identify the music video playing.
echo "<h1 align=\"center\">Music Box:<br>".$songtitle." by ".$songartist."</h1>\n";
play a video
This is the embed code for a YouTube video, using the YouTube video code we stored.
This is set to autoplay. Autoplay is normally considered impolite on a web site because people might be at work or school or other setting where sudden blaring of sound or music would disturb others, but in this case the visitor intent is to listen to music, without having to push a play button for every single song.
Strings use double quotes ("
) because we use escape characters and want those interpretted.
echo "<p align=\"center\"><object style=\"height: 390px; width: 640px\"><param name=\"movie\" value=\"http://www.youtube.com/v/".$youtubecode."?version=3&autoplay=1&rel=0&feature=player_embedded\"><param name=\"allowFullScreen\" value=\"true\"><param name=\"allowScriptAccess\" value=\"always\"><embed src=\"http://www.youtube.com/v/".$youtubecode."?version=3&autoplay=1&rel=0&feature=player_embedded\" type=\"application/x-shockwave-flash\" allowfullscreen=\"true\" allowScriptAccess=\"always\" width=\"640\" height=\"360\"></object>\n";
description
The YouTube Terms of Service require that web pages with embedded videos must add context. The purpose of the description line os to add useful information that both meets this requirement and also provides your listeners with something interesting to read.
The YouTube Terms of Service forbid most commercial material (including banner ads) from web pages with embedded videos. So, we avoid placing any ads on the web page, which is an important part of the appeal fo this player anyway (no ads, no cost, all legal).
echo "<p align=\"left\"> ".$description."</p>\n";
footer
We switch back from PHP code to HTML to give the footer to end the web page.
You can follow the link to the full customizable music player (still being built). I will be providing the entire source code of the full music player, along with instruction.
The following web pages will build up from the simple player to the full version. Feel free to customize and play with the source code and personalize your own version (as long as it is for non-commerical and/or educational and/or non-profit and/or personal use).
I do ask that you give copyright credit for my original source ccde and a link to either this web site or the actual music player web site (or even better, both).
Download all the items needed for this simple player. Remember to change the name of simple.txt to simple.php (I had to rename it so that it would download rather than just run).
contact
comments, suggestions, corrections, criticisms
Names and logos of various OSs are trademarks of their respective owners.